11:30:27
Mybatis+mysql 入门使用
Mybatis+mysql 配置文件版本入门使用
Mybatis+mysql 注解版本入门使用
一、新建一个java maven项目-Mybatis+mysql 配置文件版本入门使用
groupId:cn.zc.javapro.database
artifactId:SimpleMybatisMysql
version:1.0-SNAPSHOT
,目录结构如下所示:
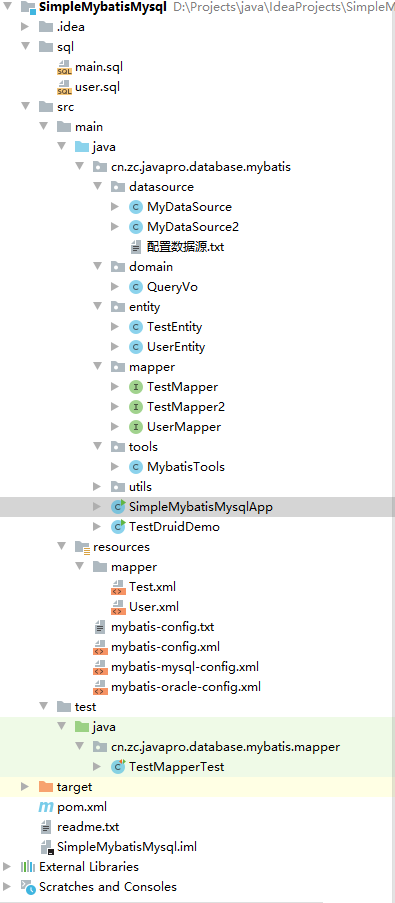
1、添加依赖
a)使用maven管理依赖,以我本次使用的版本为例

4.0.0
cn.zc.javapro.database
SimpleMybatisMysql
1.0-SNAPSHOT
UTF-8
1.7
1.7
junit
junit
4.12
test
org.mybatis
mybatis
3.5.6
mysql
mysql-connector-java
8.0.13
runtime
com.alibaba
druid
1.1.16
org.projectlombok
lombok
1.18.16
org.apache.maven.plugins
maven-compiler-plugin
8
8
pom.xml
b) 也可以使用gradle管理依赖
c) 也可以去官网下载,mybatis-3官网地址:http://www.mybatis.org/mybatis-3/
2、初始化数据库和初始数据
以数据库db2021和表t_test为例,以下是sql。然后执行sql,经此步骤,数据库中存在数据库db2021和表t_test
-- 2、初始化数据库和初始数据,以数据库moy_mybatis和表t_test为例
-- 创建数据库
CREATE SCHEMA IF NOT EXISTS `db2021` Character Set utf8mb4 COLLATE utf8mb4_unicode_ci;
USE `db2021`;
-- 创建一个测试表
CREATE TABLE IF NOT EXISTS `db2021`.`t_test`(
id INT(11) AUTO_INCREMENT,
create_time DATE COMMENT '创建时间',
modify_time DATE COMMENT '修改时间',
content VARCHAR (50) COMMENT '内容',
PRIMARY KEY (id)
);
3、新建实体类TestEntity.java
为了接收从表中取出来的值,比如数据库的select语句返回来的值,我们通过实体类对象进行接收。所以需要定义表的实体类。
关键技术点是Mybatis如何实现封装,如何实现表列和实体类对象成员之间的一一映射。

package cn.zc.javapro.database.mybatis.entity;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import java.io.Serializable;
import java.util.Date;
/**
* [Project]:
* [Date]:2021/1/22
* [Description]: java mybatis mysql maven
* @author zc
*/
//让它实现序列化接口
@Getter
@Setter
@ToString
public class TestEntity implements Serializable {
private Integer id;
private Date createTime;
private Date modifyTime;
private String content;
}
TestEntity.java
这里我们可以玩个小花样,再用个实体类QueryVo封装一下TestEntity实体类。

package cn.zc.javapro.database.mybatis.domain;
import cn.zc.javapro.database.mybatis.entity.TestEntity;
public class QueryVo {
private TestEntity testEntity;
public TestEntity getTestEntity() {
return testEntity;
}
public void setTestEntity(TestEntity testEntity) {
this.testEntity = testEntity;
}
}
QueryVo.java
4、新建接口TestMapper.java
Mybatis通过定义接口,并通过配置文件或者注解,自动实现将该接口中的方法与sql语句一一对应。
当调用该接口的方法,相当于调用了对应的sql语句。

package cn.zc.javapro.database.mybatis.mapper;
import cn.zc.javapro.database.mybatis.domain.QueryVo;
import cn.zc.javapro.database.mybatis.entity.TestEntity;
import org.apache.ibatis.annotations.Param;
import java.io.Serializable;
import java.util.List;
/**
* [Project]:
* [Email]:
* [Date]:2021/1/22
* [Description]:
*
* @author zc
*/
public interface TestMapper {
List list();
TestEntity get(Serializable id);
//TestEntity get(Integer id);
//TestEntity get(@Param("id") Integer id);
int insert(TestEntity TestEntity);
int update(TestEntity TestEntity);
int delete(Serializable id);
int count();
/**
* 根据名称模糊查询信息
*/
List findByContent(String content);
/**
* 根据queryVo中的条件查询用户
*/
List findTestByVo(QueryVo vo);
}
TestMapper.java
5、 新建实体映射文件Test.xml
这里主要配置 接口类的方法 与 sql语句一一对应关系。比如, TestMapper 与 TestEntity、QueryVo等。
这里的namespace就是写TestMapper全类名,否则不能使用动态代理的方式调用接口TestMapper。后面会讲一下parameterType如何配置多个参数。

SELECT * FROM t_test
SELECT count(*) FROM t_test
SELECT id,create_time as createTime,modify_time as modifyTime, content
FROM t_test WHERE id=#{id}
INSERT INTO t_test (create_time,modify_time,content)
VALUES (#{createTime},#{modifyTime},#{content})
UPDATE t_test SET modify_time=#{modifyTime},content=#{content}
WHERE id = #{id}
DELETE FROM t_test WHERE id = #{id}
select id, create_time as createTime, modify_time as modifyTime, content
from t_test where content like '%${content}%'
select id, create_time as createTime, modify_time as modifyTime, content
from t_test where id like #{testEntity.id};
Test.xml
6、新建mybatis配置信息文件mybatis-config.xml
这里主要配置项目实体类对应的包名,可以扫描实体类。并配置了mysql数据库的连接信息。以及配置了 接口类与实体类映射的配置文件(这里例如Test.xml)的路径。

DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
mybatis-config.xml
这里重点说一下,我们可以单独通过XML来配置环境变量。
也可以通过 xml + properties 文件进行配置,
也可以通过 properties + xml文件进行配置,
还可以通过 yml + xml 文件进行配置。
参考: SpringBoot配置Mybatis的两种方式(通过XML配置和通过YML配置文件配置): https://blog.csdn.net/weixin_43966635/article/details/112342116
比如,xml的配置还有另一种写法,即 xml + properties,下面的只写主要部分:

1.内置连接池
2.引入数据源
mybatis-config
7、为了方便测试,编写一个获取SqlSession的工具类MybatisTools.java
该类主要通过配置文件(如,mybatis-config.xml)初始化 SqlSession,通过SqlSession 配置注册初始化 接口类(如 TestMapper)

package cn.zc.javapro.database.mybatis.tools;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.ExecutorType;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException;
import java.io.Reader;
import java.util.Objects;
/**
* [Project]:
* [Email]:
* [Date]:2021/1/22
* [Description]:
* 7、为了方便测试,编写一个获取SqlSession的工具类MybatisTools
* 该类主要是加载mybatis的配置文件(如:mybatis-config.xml等),然后
*
* 使用的时候:
* 1)先 MybatisTools mt = new MybatisTools(resourceXMLFile) // 这里的resourceXMLFile是mybatis的配置文件,如"mybatis-config.xml"
* 2) SqlSession sqlSession = mt.openCurrentSqlSession() // 按照配置文件打开获取 SqlSession
* 3) TestMapper testMapper = sqlSession.getMapper(TestMapper.class); // 这里的 TestMapper.class 是实体和数据库的交互接口。
* 4) testMapper.getAll() // 调用方法
* 5) mt.closeCurrentSqlSession() // 关闭 SqlSession
* @author zc
*/
public class MybatisTools {
//private String resourceFile;
private ExecutorType executorType;
private SqlSessionFactory sqlSessionFactory;
private ThreadLocal sessionThread = new ThreadLocal();
/**
*
* @param resourceFile 资源文件
*/
public MybatisTools(String resourceFile) {
//this.resourceFile = resourceFile;
this.initSqlSessionFactory(resourceFile);
}
/**
*
* @param resourceFile
* @param executorType
*/
public MybatisTools(String resourceFile, ExecutorType executorType) {
//this.resourceFile = resourceFile;
this.initSqlSessionFactory(resourceFile);
this.executorType = executorType;
}
private void initSqlSessionFactory(String resourceFile) {
try {
Reader reader = Resources.getResourceAsReader(resourceFile);
// 构建sqlSession的工厂
sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public SqlSession openCurrentSqlSession() {
SqlSession sqlSession = sessionThread.get();
if (Objects.isNull(sqlSession)) {
if(this.executorType == null) {
sqlSession = sqlSessionFactory.openSession();
} else {
// 通过session设置ExecutorType开启批量添加,类似jdbc的addBatch操作
//sqlSession = sqlSessionFactory.openSession(ExecutorType.BATCH);
sqlSession = sqlSessionFactory.openSession(this.executorType);
}
sessionThread.set(sqlSession);
}
return sqlSession;
}
public void commitCurrentSqlSession() {
SqlSession sqlSession = sessionThread.get();
if (Objects.nonNull(sqlSession)) {
sqlSession.commit();
}
}
public void closeCurrentSqlSession() {
SqlSession sqlSession = sessionThread.get();
if (Objects.nonNull(sqlSession)) {
/*if (this.executorType != null) {
sqlSession.commit();
}*/
sqlSession.close();
}
sessionThread.set(null);
}
}
MybatisTools.java
执行查询语句的时候不需要commit,但是执行 insert、update等操作时,需要commit。并且如果是大批量的更新插入操作,则每次都打开关闭,会浪费。所以我们直接一次插入。具体看第八节。
8、 新建测试类TestMapperTest测试
包括3步:开启SqlSession;执行接口类的方法(如TestMapper的get()方法 );关闭SqlSession。

package cn.zc.javapro.database.mybatis.mapper;
import cn.zc.javapro.database.mybatis.domain.QueryVo;
import cn.zc.javapro.database.mybatis.entity.TestEntity;
import cn.zc.javapro.database.mybatis.tools.MybatisTools;
import cn.zc.javapro.database.mybatis.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import java.util.Arrays;
import java.util.Date;
import java.util.List;
public class TestMapperTest {
MybatisTools mybatisTools;
SqlSession sqlSession;
TestMapper testMapper;
@Before
public void before() {
/*sqlSession = MybatisUtils.getCurrentSqlSession();
testMapper = sqlSession.getMapper(TestMapper.class);*/
mybatisTools = new MybatisTools("mybatis-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
testMapper = sqlSession.getMapper(TestMapper.class);
}
@After
public void after() {
// MybatisUtils.closeCurrentSession();
mybatisTools.closeCurrentSqlSession();
}
@Test
public void insert() {
TestEntity entity = new TestEntity();
entity.setCreateTime(new Date());
entity.setModifyTime(new Date());
entity.setContent("我是内容");
//entity.setaontent("我是内容");
System.out.println(testMapper.insert(entity));
sqlSession.commit();
}
@Test
public void count() {
System.out.println(testMapper.count());
}
@Test
public void list() {
List list = testMapper.list();
//System.out.println(Arrays.toString(list.toArray()));
for (TestEntity testEntity : list) {
System.out.println(testEntity);
}
}
@Test
public void get() {
System.out.println(testMapper.get(1));
}
@Test
public void update() {
TestEntity entity = new TestEntity();
entity.setId(1);
entity.setModifyTime(new Date());
entity.setContent("我是修改后内容");
//entity.setaontent("我是修改后内容");
testMapper.update(entity);
sqlSession.commit();
}
@Test
public void delete() {
testMapper.delete(1);
sqlSession.commit();
}
/**
* 测试模糊查询操作
*/
@Test
public void findByContent(){
List testEntityList = testMapper.findByContent("内容");
for(TestEntity testEntity: testEntityList){
System.out.println(testEntity);
}
}
/**
* 测试使用QueryVo作为查询对象
*/
@Test
public void testFindByVo(){
QueryVo vo = new QueryVo();
TestEntity testEntity = new TestEntity();
//testEntity.setContent("%内容%");
testEntity.setId(1);
vo.setTestEntity(testEntity);
List testEntityList = testMapper.findTestByVo(vo);
for(TestEntity testEntity1 : testEntityList){
System.out.println(testEntity1);
}
}
}
TestMapperTest
我们可以看到,已经可以操作了。下面我们试一下主方法中调用:
9、主方法中调用
包括3步:开启SqlSession;执行接口类的方法(如TestMapper的get()方法 );关闭SqlSession。

package cn.zc.javapro.database.mybatis;
import cn.zc.javapro.database.mybatis.mapper.TestMapper;
import cn.zc.javapro.database.mybatis.tools.MybatisTools;
import cn.zc.javapro.database.mybatis.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
public class SimpleMybatisMysqlApp {
public static void main(String[] args) {
test2();
}
public static void test2() {
SqlSession sqlSession;
TestMapper testMapper;
//
MybatisTools mybatisTools = new MybatisTools("mybatis-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
//得到 TestMapper 接口的实现类对象,TestMapper 接口的实现类对象由 sqlSession.getMapper(TestMapper.class)动态构建出来
testMapper = sqlSession.getMapper(TestMapper.class);
//执行查询操作,将查询结果自动封装成 TestEntity 返回
System.out.println(testMapper.get(1));
mybatisTools.closeCurrentSqlSession();
}
public static void test() {
SqlSession sqlSession;
TestMapper testMapper;
sqlSession = MybatisUtils.getCurrentSqlSession();
testMapper = sqlSession.getMapper(TestMapper.class);
//
System.out.println(testMapper.get(1));
MybatisUtils.closeCurrentSession();
}
}
SimpleMybatisMysqlApp.java
可以看出来,依然可以得出结果。到这一步,我们已经可以使用mybatis对数据库进行操作了。
二、Mybatis (ParameterType) 如何传递多个不同类型的参数
偶然碰到一个需要给xml传一个String类型和一个Integer类型的需求,当时心想用map感觉有点太浪费,所以专门研究了下各种方式。
1、 方法一:不需要写parameterType参数
public List getXXXBeanList(String xxId, String xxCode);
select t.* from tableName where id = #{0} and name = #{1}
由于是多参数那么就不能使用parameterType, 改用#{index}是第几个就用第几个的索引,索引从0开始
2、方法二:基于注解(最简单)
public List getXXXBeanList(@Param("id")String id, @Param("code")String code);
select t.* from tableName where id = #{id} and name = #{code}
由于是多参数那么就不能使用parameterType, 这里用@Param来指定哪一个
3、方法三:Map封装
public List getXXXBeanList(HashMap map);
select 字段... from XXX where id=#{xxId} code = #{xxCode}
其中hashmap是mybatis自己配置好的直接使用就行。map中key的名字是那个就在#{}使用那个,map如何封装就不用了我说了吧。
4、方法四:List封装
public List getXXXBeanList(List list);
select 字段... from XXX where id in
#{item}
5、总结
传递list和map在资源消耗上肯定远大于方法一和方法二,但是有一些特殊的情形需要传递list,比如你需要传递一个id集合并批量对id进行sql操作然后再返回等等。所以都需要了解。
四、Mybatis (resultType) 返回结果
如何对应实体类的成员变量,之前说过我们实体类的属性名和数据库列名是一致的,那如果是不一致的呢。
因为MySQL数据库不区分大小写,userName和username等同所以能封装进去,而其他的完全不一样,就封装不进去。
这里注意如果是linux系统是严格区分大小写的。
现在我们来解决封装不进去的问题,之所以封装不进去就是名字对不上,我们可以通过起别名的方式解决。
有2种解决办法:都是通过配置 Tese.xml .
1、通过起别名的方式
现在我们来解决封装不进去的问题,之所以封装不进去就是名字对不上,我们可以通过起别名的方式解决。
也就是说mysql执行这条sql语句出来的列名跟实体类是需要匹配的。如下所示:我们只是截取Test.xml中的片段。

SELECT id,create_time as createTime,modify_time as modifyTime ,content
FROM t_test
SELECT id,create_time as createTime,modify_time as modifyTime ,content
FROM t_test WHERE id=#{id}
INSERT INTO t_test (create_time,modify_time,content)
VALUES (#{createTime},#{modifyTime},#{content})
UPDATE t_test SET modify_time=#{modifyTime},content=#{content}
WHERE id = #{id}
DELETE FROM t_test WHERE id = #{id}
Test.xml片段
我们可以看到select 、insert、update、delete 都是将表列名和实体成员变量名对应起来。
如果我们用了
SELECT * FROM t_test
就会出现id和content有内容,而 createTime 和 modifyTime为 null。这是因为id和content表列名和对象成员变量一致,而表中create_time ,成员变量createTime ;表中modify_time,成员变量modifyTime名字不一致,Mybatis无法将之对应起来,无法赋值。
同理 insert、update、delete。
2、文档进行一个配置,创建了一个结果集的关系表
创建了一个结果集的关系表,使得java实体类的属性名和数据库的列名一一对应,property是java属性名,column是表的列名。
我们截取了Test.xml片段

DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
SELECT * FROM t_test
SELECT * FROM t_test WHERE id=#{id}
Test.xml
这样就可以将表列名和对象成员变量一一对应起来。
总结
第一种方式是直接在数据库层实现,第二种方式还要多解析个xml,所以第一种的执行效率比第二种的要高,但第二种的开发效率高,因为我们既然定义了这个map,之后有关查询的都可以配置这个map,不用动里面的sql语句,两种方法各有利弊。
五、Mybatis+mysql 注解版本入门使用
只需要简单的修改就可以了,首先注解版本的实现就是用注解代替了Test.xml,所以注解版本不需要Test.xml。
1、修改 mybatis-config.xml

DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
mybatis-config.xml
主要是修改了:
2、添加接口 TestMapper2.java
这里面就是注解的实现。

package cn.zc.javapro.database.mybatis.mapper;
import cn.zc.javapro.database.mybatis.domain.QueryVo;
import cn.zc.javapro.database.mybatis.entity.TestEntity;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
import java.io.Serializable;
import java.util.List;
/**
* [Project]:
* [Email]:
* [Date]:2021/1/22
* [Description]:
*
* @author zc
*/
public interface TestMapper2 {
@Select("select id, create_time as createTime, modify_time as modifyTime, content from t_test;")
List list();
@Select("select id, create_time as createTime, modify_time as modifyTime, content from t_test where id = #{id};")
TestEntity get(Serializable id);
//TestEntity get(Integer id);
//TestEntity get(@Param("id") Integer id);
@Insert("INSERT INTO t_test (create_time,modify_time,content)\n" +
" VALUES (#{createTime},#{modifyTime},#{content})")
int insert(TestEntity TestEntity);
@Update("UPDATE t_test SET modify_time=#{modifyTime},content=#{content}\n" +
" WHERE id = #{id}")
int update(TestEntity TestEntity);
@Delete("DELETE FROM t_test WHERE id = #{id}")
int delete(Serializable id);
@Select("SELECT count(*) FROM t_test")
int count();
/**
* 根据名称模糊查询信息
*/
@Select("select id, create_time as createTime, modify_time as modifyTime, content\n" +
" from t_test where content like '%${content}%'")
List findByContent(String content);
/**
* 根据queryVo中的条件查询用户
*/
@Select("select id, create_time as createTime, modify_time as modifyTime, content\n" +
" from t_test where id like #{testEntity.id};")
List findTestByVo(QueryVo vo);
}
TestMapper2.java
3、修改主类SimpleMybatisMysqlApp,测试一下

package cn.zc.javapro.database.mybatis;
import cn.zc.javapro.database.mybatis.mapper.TestMapper;
import cn.zc.javapro.database.mybatis.mapper.TestMapper2;
import cn.zc.javapro.database.mybatis.tools.MybatisTools;
import cn.zc.javapro.database.mybatis.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
public class SimpleMybatisMysqlApp {
public static void main(String[] args) {
test3();
}
public static void test3() {
SqlSession sqlSession;
TestMapper2 testMapper2;
//
MybatisTools mybatisTools = new MybatisTools("mybatis-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
//得到 TestMapper 接口的实现类对象,TestMapper 接口的实现类对象由 sqlSession.getMapper(TestMapper.class)动态构建出来
testMapper2 = sqlSession.getMapper(TestMapper2.class);
//执行查询操作,将查询结果自动封装成 TestEntity 返回
System.out.println(testMapper2.get(1));
mybatisTools.closeCurrentSqlSession();
}
public static void test2() {
SqlSession sqlSession;
TestMapper testMapper;
//
MybatisTools mybatisTools = new MybatisTools("mybatis-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
//得到 TestMapper 接口的实现类对象,TestMapper 接口的实现类对象由 sqlSession.getMapper(TestMapper.class)动态构建出来
testMapper = sqlSession.getMapper(TestMapper.class);
//执行查询操作,将查询结果自动封装成 TestEntity 返回
System.out.println(testMapper.get(1));
mybatisTools.closeCurrentSqlSession();
}
public static void test() {
SqlSession sqlSession;
TestMapper testMapper;
sqlSession = MybatisUtils.getCurrentSqlSession();
testMapper = sqlSession.getMapper(TestMapper.class);
//
System.out.println(testMapper.get(1));
MybatisUtils.closeCurrentSession();
}
}
SimpleMybatisMysqlApp.java
六、自定义的数据源
自定义数据源有两种写法;之后需要修改一下 mybatis-config.xml,使得Mybatis识别使用该数据源。
1、我们配置一下自定义的数据源
比如我们自定义数据源整合阿里巴巴的druid数据库连接池:
方案一:extends DruidDataSourceFactory implements DataSourceFactory
方案二:extends UnpooledDataSourceFactory
方案一:MyDataSource.java

package cn.zc.javapro.database.mybatis.datasource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import org.apache.ibatis.datasource.DataSourceFactory;
import javax.sql.DataSource;
import java.util.Properties;
public class MyDataSource extends DruidDataSourceFactory implements DataSourceFactory {
protected Properties properties;
@Override
public void setProperties(Properties properties) {
this.properties= properties;
}
@Override
public DataSource getDataSource() {
try {
return createDataSource(properties);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
}
MyDataSource.java
方案二:MyDataSource2.java

package cn.zc.javapro.database.mybatis.datasource;
import com.alibaba.druid.pool.DruidDataSource;
import org.apache.ibatis.datasource.unpooled.UnpooledDataSourceFactory;
import javax.sql.DataSource;
public class MyDataSource2 extends UnpooledDataSourceFactory {
protected DataSource dataSource;
public MyDataSource2() {
this.dataSource = new DruidDataSource();
}
}
MyDataSource2.java
2、修改mybatis-config.xml,如下所示:

DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
mybatis-config.xml
3、编写测试方法TestDruidDemo.java

package cn.zc.javapro.database.mybatis;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.Reader;
public class TestDruidDemo {
/**
* @param args
* @throws Exception
*/
public static void main(String[] args) throws Exception {
String resource = "mybatis-config.xml";
// 使用类加载器加载mybatis的配置文件(它也加载关联的映射文件)
Reader reader = Resources.getResourceAsReader(resource);
// 构建sqlSession的工厂
SqlSessionFactory sessionFactory = new SqlSessionFactoryBuilder()
.build(reader,"development");
SqlSession session = sessionFactory.openSession();
System.out.println(session);
session = sessionFactory.openSession();
System.out.println(session);
DruidDataSourceFactory f;
}
}
TestDruidDemo.java
七、配置多数据源
配置多数据源(此数据源非比数据源,此处更偏向于数据库,或者数据的来源),就是通过不同的配置从不同的数据源中获取数据。比如MySQL的主库和从库(或者读库和写库),不是一个库,需要配置两个数据源。甚至是不同数据库的数据源,有的来自MySQL、有的来自Oracle等,都需要配置多数据源。
具体如下,我们还是在之前的基础上进行修改。
比如,我现在要从另一个数据库获取数据,这是我就要重新配置一个新的mybatis-mysql-config.xml,他和mybatis-config.xml同一级别,知识不同的配置。
1、配置一个新的mybatis-mysql-config.xml,因为来自不同的库。

DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
mybatis-mysql-config.xml
2、添加一个新的实体类UserEntity.java

package cn.zc.javapro.database.mybatis.entity;
import lombok.Data;
import java.io.Serializable;
@Data
public class UserEntity implements Serializable {
private int id;
// 邮箱
private String email;
// 昵称
private String nickName;
// 密码
private String passWord;
// 注册时间
private String regTime;
// 用户名
private String userName;
}
UserEntity.java
3、添加一个新的Mapper,UserMapper.java

package cn.zc.javapro.database.mybatis.mapper;
import cn.zc.javapro.database.mybatis.entity.UserEntity;
import java.util.List;
public interface UserMapper {
List list();
}
UserMapper.java
4、添加一个新的配置文件,User.xml

DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
SELECT * FROM user
User.xml
5、修改主类,进行测试

package cn.zc.javapro.database.mybatis;
import cn.zc.javapro.database.mybatis.entity.UserEntity;
import cn.zc.javapro.database.mybatis.mapper.TestMapper;
import cn.zc.javapro.database.mybatis.mapper.TestMapper2;
import cn.zc.javapro.database.mybatis.mapper.UserMapper;
import cn.zc.javapro.database.mybatis.tools.MybatisTools;
import cn.zc.javapro.database.mybatis.utils.MybatisUtils;
import org.apache.ibatis.session.SqlSession;
public class SimpleMybatisMysqlApp {
public static void main(String[] args) {
System.out.println("------------test3--------------");
test3();
System.out.println("------------test4--------------");
test4();
}
public static void test4() {
SqlSession sqlSession;
UserMapper userMapper;
//
MybatisTools mybatisTools = new MybatisTools("mybatis-mysql-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
//得到 UserMapper 接口的实现类对象,UserMapper 接口的实现类对象由 sqlSession.getMapper(UserMapper.class)动态构建出来
userMapper = sqlSession.getMapper(UserMapper.class);
//执行查询操作,将查询结果自动封装成 UserEntity 返回
for(UserEntity userEntity: userMapper.list()) {
System.out.println(userEntity);
}
mybatisTools.closeCurrentSqlSession();
}
public static void test3() {
SqlSession sqlSession;
TestMapper2 testMapper2;
//
MybatisTools mybatisTools = new MybatisTools("mybatis-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
//得到 TestMapper 接口的实现类对象,TestMapper 接口的实现类对象由 sqlSession.getMapper(TestMapper.class)动态构建出来
testMapper2 = sqlSession.getMapper(TestMapper2.class);
//执行查询操作,将查询结果自动封装成 TestEntity 返回
System.out.println(testMapper2.get(1));
mybatisTools.closeCurrentSqlSession();
}
public static void test2() {
SqlSession sqlSession;
TestMapper testMapper;
//
MybatisTools mybatisTools = new MybatisTools("mybatis-config.xml");
sqlSession = mybatisTools.openCurrentSqlSession();
//得到 TestMapper 接口的实现类对象,TestMapper 接口的实现类对象由 sqlSession.getMapper(TestMapper.class)动态构建出来
testMapper = sqlSession.getMapper(TestMapper.class);
//执行查询操作,将查询结果自动封装成 TestEntity 返回
System.out.println(testMapper.get(1));
mybatisTools.closeCurrentSqlSession();
}
public static void test() {
SqlSession sqlSession;
TestMapper testMapper;
sqlSession = MybatisUtils.getCurrentSqlSession();
testMapper = sqlSession.getMapper(TestMapper.class);
//
System.out.println(testMapper.get(1));
MybatisUtils.closeCurrentSession();
}
}
SimpleMybatisMysqlApp.java
结果如下:
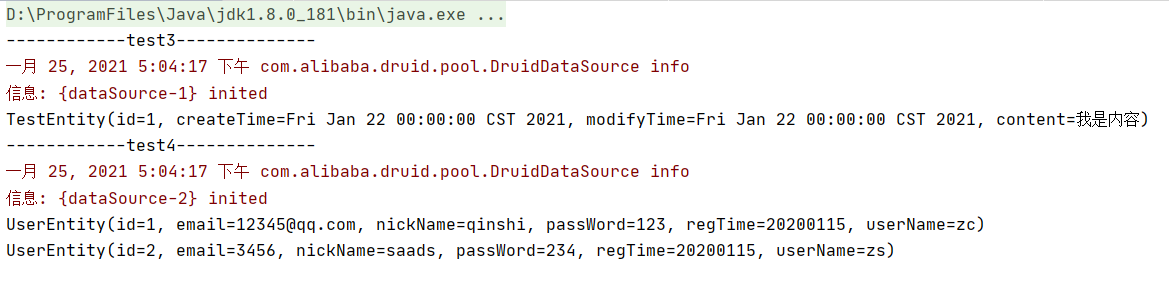
八、批量插入Insert与批量更新Update
待补充
https://www.cnblogs.com/zhangchao0515/p/14333262.html
参考:
Mybatis中进行批量更新(updateBatch):https://www.cnblogs.com/eternityz/p/12284760.html
Mybatis批量新增和修改:https://blog.csdn.net/lan_qinger/article/details/84138216
MyBatis批量新增数据:https://www.cnblogs.com/shouyaya/p/12797984.html
总结:
1) Mybatis是在 JDBC基础上进行进一步封装
2)Mybatis用到了很多设计模式,包括但不限于 工厂模式,建造者模式,代理模式,适配模式(比如MySQL、Oracle等);还用到反射等。
3)Mybatis隐藏了细节,最终肯定要把实体类的成员变量和表的列名一一对应起来。 其中实体类的成员变量通过get方法获取值,通过set方法设置值。
|